Why would you want to "read" a smart contract or interact with it?
You can find detailed data on chain when you "read" smart contracts. You can "write" smart contracts to change data in them. Having the ability to interact with smart contracts directly gives you a big advantage in web3.
Smart contracts are not business contracts. They are programs running on the blockchain. As cantino.eth says in a tweet thread, reading the smart contract code of a project “gives you insight into the popularity of a project, how distributed its ownership is, and what it’s capable of”.
Let’s begin interacting with smart contracts using this 5 part how-to guide:
- What is a smart contract?
- Read general information
- Read transaction details
- Read from a contract: Query data from a contract
- Writing to a contract: Call state-transition functions
1. What is a smart contract?
1.1 Smart contracts
A "smart contract" is simply a program that runs on the Ethereum blockchain.
It's a collection of code (its functions) and data (its state) that resides at a specific address on the Ethereum blockchain.
Every token(fungible or non-fungible) is backed by a smart contract. For example, the Boring Ape Yacht Club(BAYC) NFT is based on a ERC721-standard smart contract on Ethereum blockchain.
1.2 Understanding the blockchain explorer
Follow the contract address link to explore the BAYC ERC-721 smart contract on Etherscan: https://etherscan.io/address/0xbc4ca0eda7647a8ab7c2061c2e118a18a936f13d . Etherscan is a blockchain explorer of Ethereum.
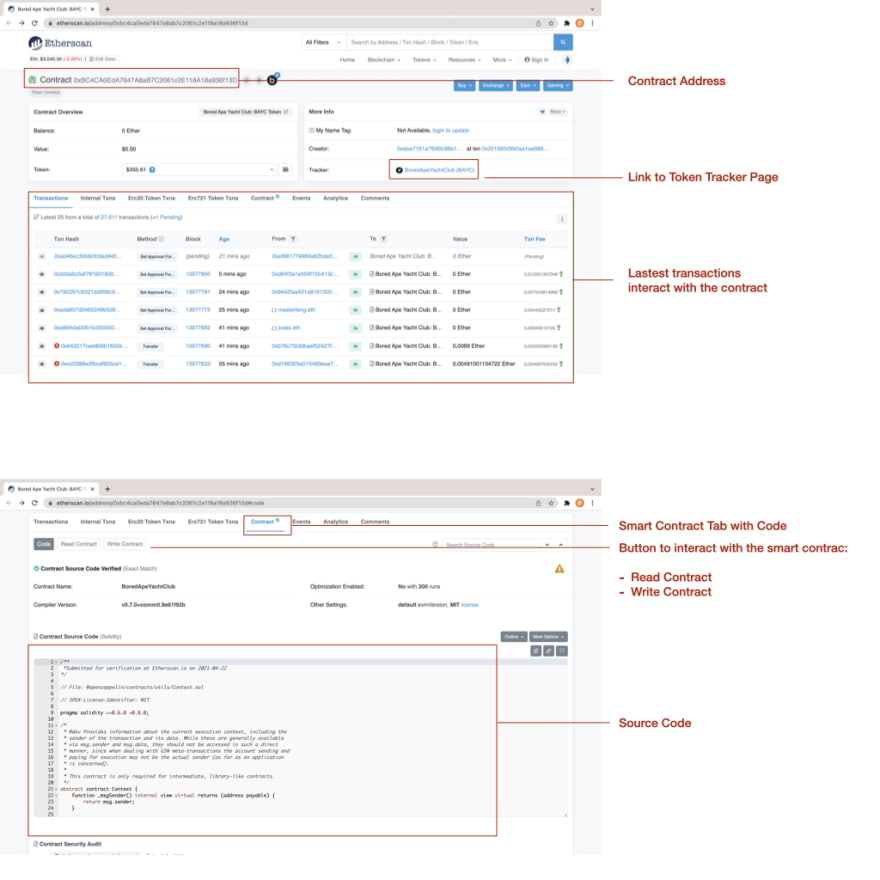
On Etherscan, we can see detailed data from the smart contract such as the latest transactions as well as its source code (if it is open source and has been validated on Etherscan).
In this context, reading a contract means that we extract data from it without altering the data stored in it. Writing to a contract means that do change the internal data, specifically by calling a smart contract function.
For ERC20 and ERC721 tokens, Etherscan provides token tracker pages that allow us to automatically parse the respective smart contracts.
Here's the token tracker page for BAYC: https://etherscan.io/token/0xbc4ca0eda7647a8ab7c2061c2e118a18a936f13d.
2. Read general information
2.1 Total Supply, Holders, Transfers
On the BAYC Token page on Etherscan, you can find general information about this NFT collection:
- Total Supply: 10, 000 BAYC
- Holders: 6,095
- Transfers: 54,828
Note that we can also do a more powerful analysis of the NFTÂ collect. On the contract page, we find find some additional useful analytics: https://etherscan.io/address/0xbc4ca0eda7647a8ab7c2061c2e118a18a936f13d#analytics.
2.2 Holders and Distribution
In the holder tab, you can see the top holder of the NFT. Click link "Token Holder Chart", we can see a pie chart of holders.
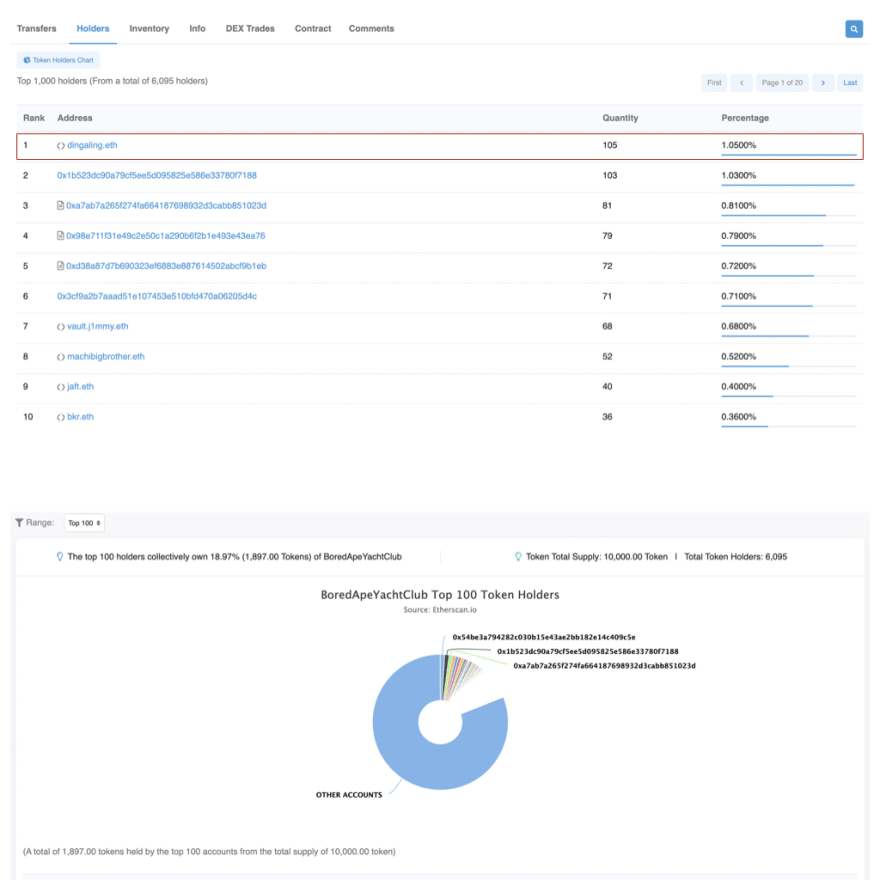
2.3 NFT Token of an address
The user dingaling.eth has 105 BAYC NFT items. Click the link of his name to open a new page and click Inventory tab, and you can see the full list of his 105 BAYC NFTs. In Etherscan, we can filter token by holder’s address.
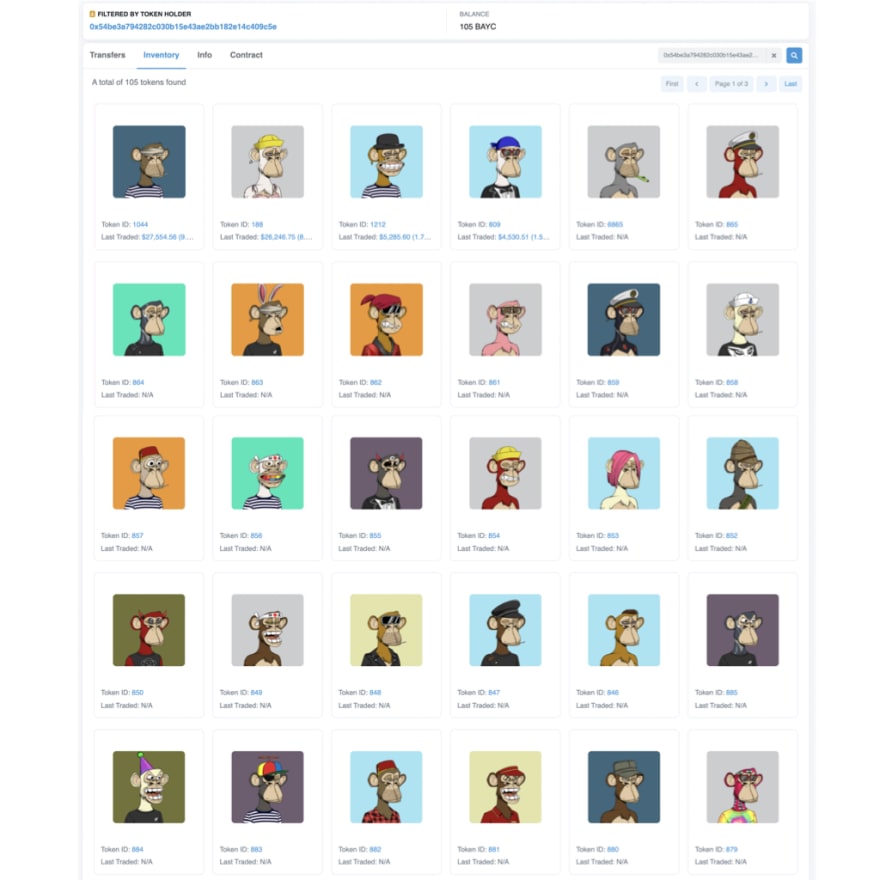
BAYC Inventory of dingaling.eth: https://etherscan.io/token/0xbc4ca0eda7647a8ab7c2061c2e118a18a936f13d?a=0x54be3a794282c030b15e43ae2bb182e14c409c5e#inventory.
3. Read transaction details
Whenever we transfer a token from one address to another, a transaction occurs on the blockchain. When we try to mint a NFT on a web page, we are also sending a transaction.
3.1 Transfer/Transactions
On the token tracker page, you can see a full list of token transactions/transfers.
There are six rows in the table:
- Transaction hash (Txn Hash)
- Method of contract we interact with (can be BAYC and others)
- Time Stamp (Age)
- Token Transfer From
- Token Transfer To
- Related Token ID

3.2 Details of a transaction
Let’s have a look at the transaction in which Stephen Curry bought BAYC #7990: https://etherscan.io/tx/0x905f9e8c58d94ca66f4d6d10d57aa612860e9b19ed08eece85862ff730494c27

We can understand this transaction by tracking the transaction details:
- BAYC #7990 is transferred from 0x399d07303275719fb83acb468249a9a26ae2f674(ArtOfBlock) to 0x3becf83939f34311b6bee143197872d877501b11(Stephen Curry) on Aug-28-2021 06:55:12 AM +UTC.
- This transaction interacted with an OpenSea contract (0x7be8076f4ea4a4ad08075c2508e481d6c946d12b) by calling its function atomicMatch_.
- By calling the contract's function atomicMatch_, the buyer pays 55 ETH($162,584.95) and the seller exchange BAYC #7990 NFT instantaneously.
4. Read from a Contract: Query data from a contract
Reading a contract means that we are querying data stored on-chain. Under the hood, we call an in-contract function which does not alter the contract's data or any blockchain state. Note that because we are not making changes to the blockchain, we don't need to connect our blockchain wallet. This means that anyone can read this type of data.
To get this a try:
Go to the contract tab and click "Read Contract" Button. https://etherscan.io/address/0xbc4ca0eda7647a8ab7c2061c2e118a18a936f13d#readContract.
One there, we can see the entire assortment of callable functions within the BAYC contract. This contract might seem familiar since it's actually based on a standard ERC-721 smart contract. If you look carefully, you'll also notice that the contract has many non-standard ERC-721 functions to add unique customizations.
You can find more about ERC-721 Standard at: https://docs.openzeppelin.com/contracts/2.x/api/token/erc721.

4.1 Stable parameters
Some of the parameters are stable. When you click related column, you can see the result directly:
- MAX_APES: 10000
- REVEAL_TIMESTAMP: 1619820000
- baseURI: ipfs://QmeSjSinHpPnmXmspMjwiXyN6zS4E9zccariGR3jxcaWtq/
- name: BoredApeYachtClub
- symbol: BAYC
- totalSupply: 10000
4.2 Query data with parameters
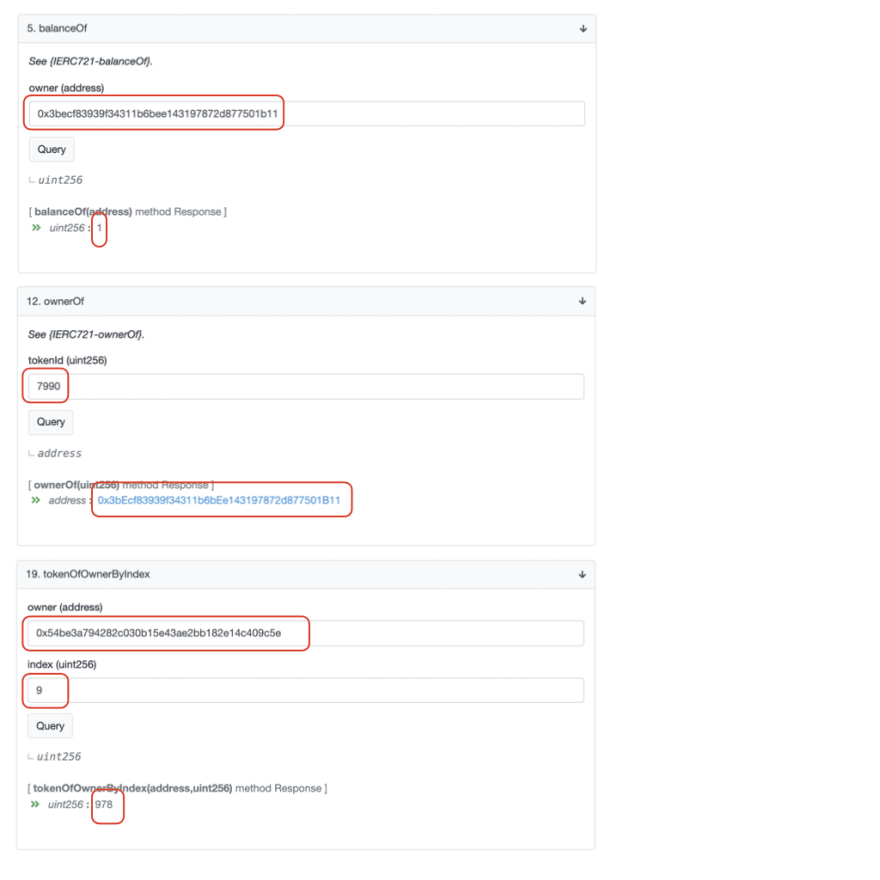
Next, let's break down a series of queries together!
- Running the balanceOf() function with Stephen Curry's Ethereum address returns 1. This make sense since Curry only has a single BAYC NFT in this wallet.
- Reading the ownerOf() with ownerOf(7990) returns 0x3becf83939f34311b6bee143197872d877501b11 which corresponds to Curry's ETHÂ address.
- Reading the function tokenOfOwnerByIndex() with tokenOfOwnerByIndex(0x54be3a794282c030b15e43ae2bb182e14c409c5e,9) returns 978. As previously explained, 0x54be3a794282c030b15e43ae2bb182e14c409c5e is the address of dingaling.eth who has 105 BAYC NFTs. This query tells us that 10th BAYC NFT is #978.
5. Writing to a contract: Call state-transition functions
While the term "writing to a contract" may invoke the idea of programming in Solidity, writing to a contract really means that we are interacting with the contract by calling state-transition functions (some may prefer to call them "methods") from our wallet. State-transition functions can be called to change the state of the smart contract and the blockchain.
5.1 Connect Wallet
While etherscan is largely a block explorer, you can treat this page of etherscan as a simple dApp. To write to a particular contract, we need to connect our wallet to this dApp.
Click “Connect to Web3”, and choose MetaMask Wallet in the popup dialog. If you choose WalletConnect, you can scan the QR code using mobile wallet app such as imToken, Rainbow, Trust Wallet, etc.
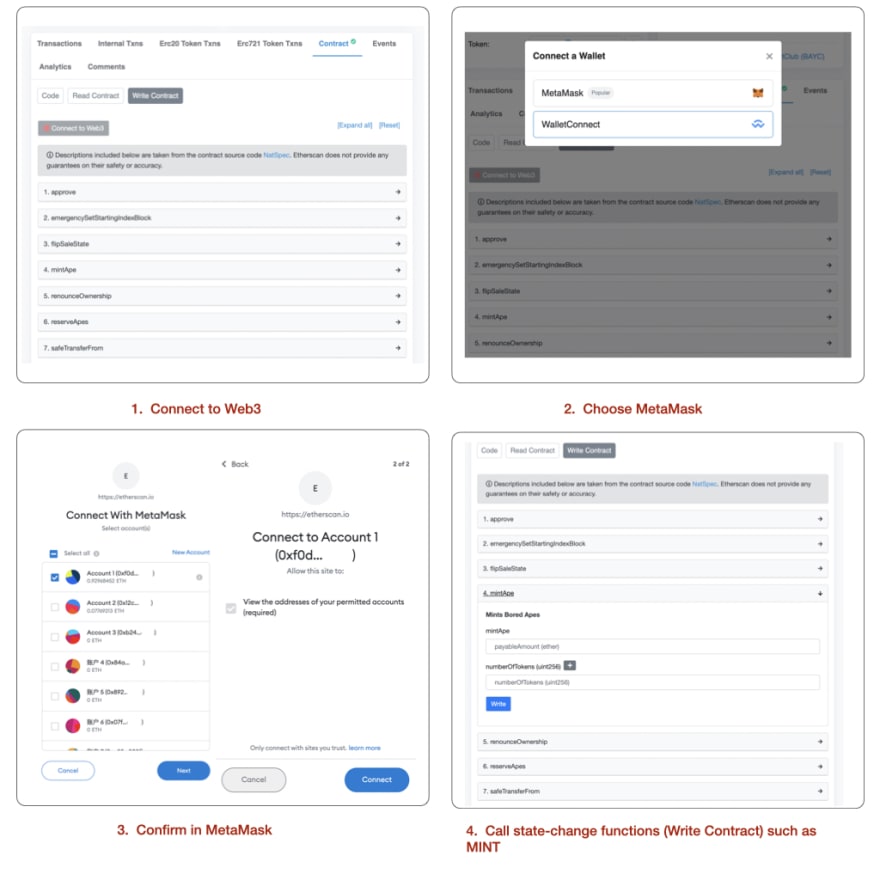
5.2 Write to Contract
Sometimes we use Etherscan's write contract feature to call state-changing functions within an NFT smart contract. The most commonly used function is mintNFT. In our example with the BAYC smart contract, the mint function is mintApe().
NFT projects usually provide a web page for users to interact with. However, under the hood, we can simply call the mint function of the smart contract directly via Etherscan.
Take Loot NFT for example. Loot and More Loot(MLoot) don't have a web page. Instead, Loot founder Dom Hoffmann asked users to mint on Etherscan. You can still mint MLoot now by calling claim(): https://etherscan.io/token/0x1dfe7ca09e99d10835bf73044a23b73fc20623df#writeContract
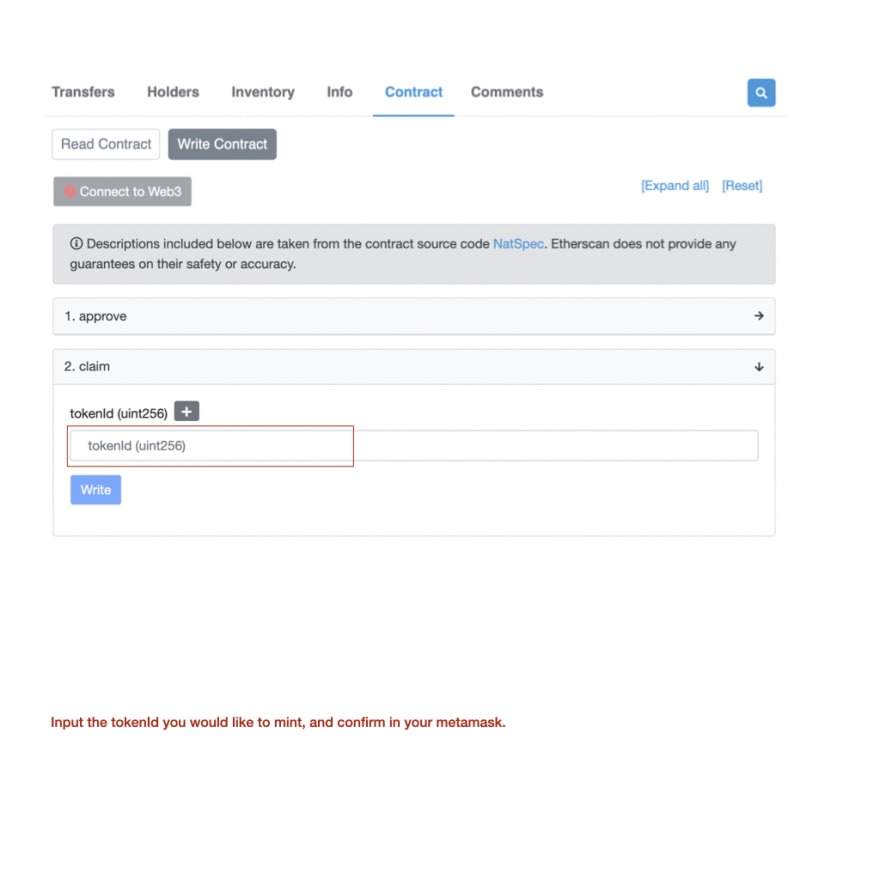
If you have NFTs in your wallet, you can do more with on Etherscan via the write contract feature. Give it a try next time and by-pass a dApp's frontend.
Following this five-part tutorial, you have now learned how to read/write (interact with) a smart contract. Now you can start your Web3 journey with this new ability. Enjoy!